





# FaceAPI
**AI-powered Face Detection & Rotation Tracking, Face Description & Recognition, Age & Gender & Emotion Prediction for Browser and NodeJS using TensorFlow/JS**
**Live Demo**:
## Additional Documentation
- [**Tutorial**](TUTORIAL.md)
- [**TypeDoc API Specification**](https://vladmandic.github.io/face-api/typedoc/index.html)
## Examples
### Browser
Browser example that uses static images and showcases both models
as well as all of the extensions is included in `/demo/index.html`
Example can be accessed directly using Git pages using URL:
Browser example that uses live webcam is included in `/demo/webcam.html`
Example can be accessed directly using Git pages using URL:
**Demo using FaceAPI to process images**
*Note: Photos shown below are taken by me*
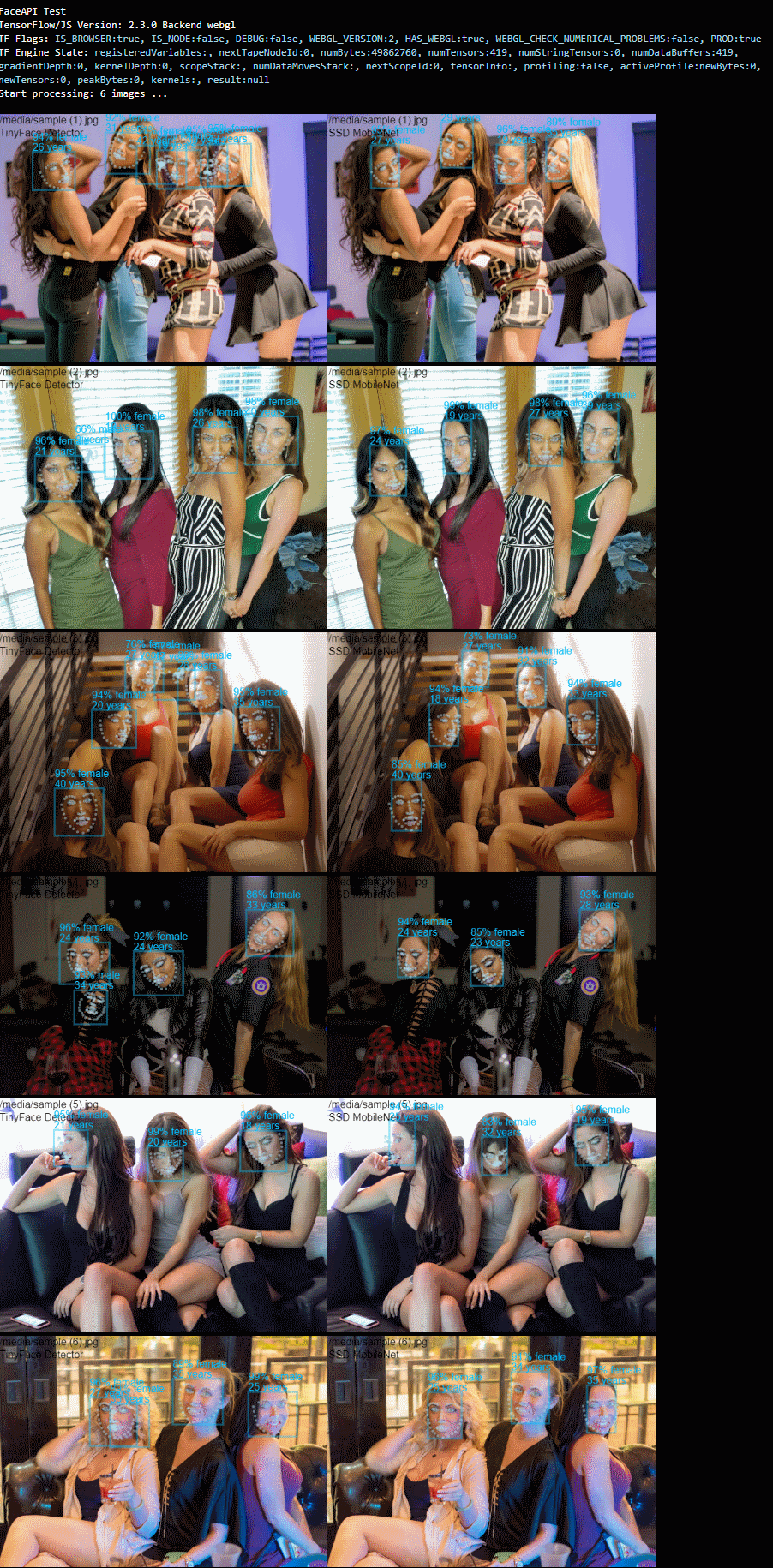
**Demo using FaceAPI to process live webcam**
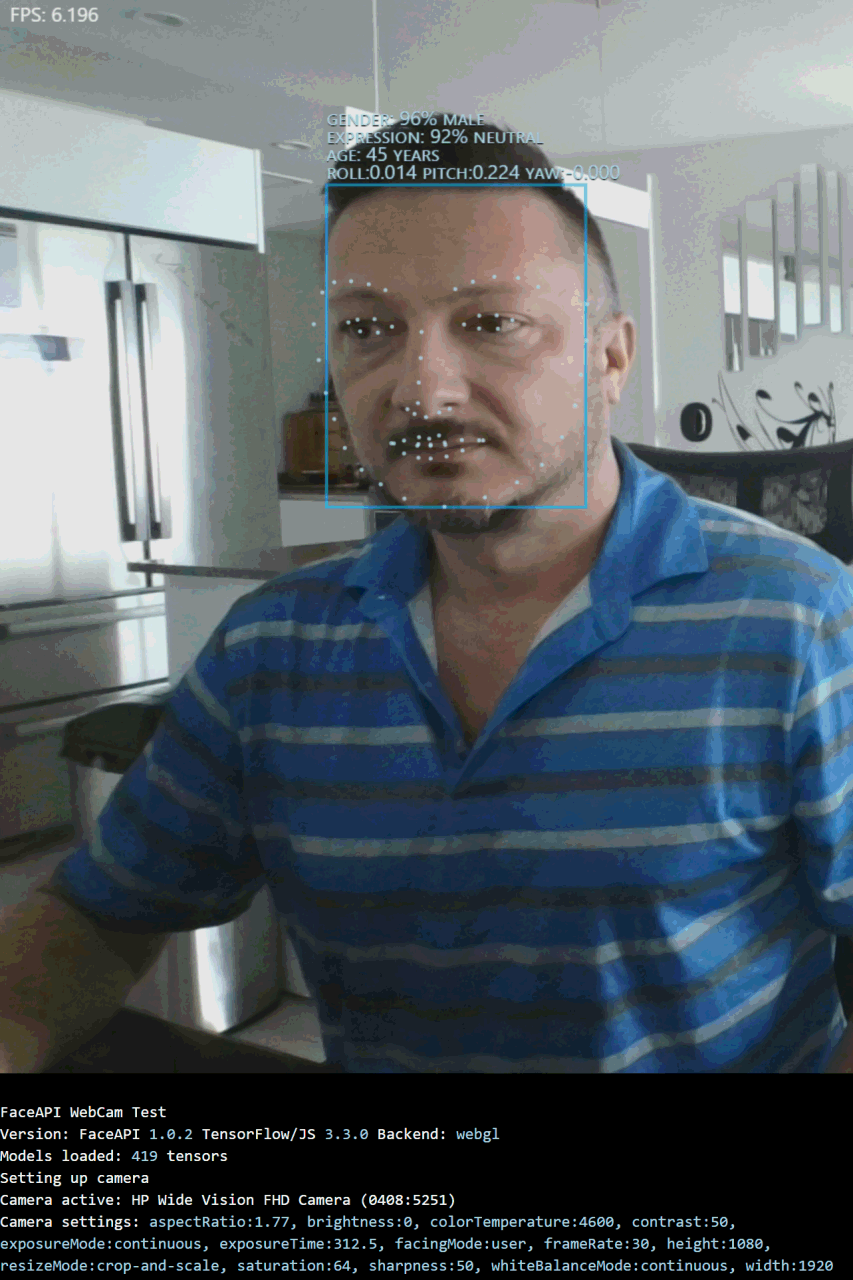
### NodeJS
NodeJS examples are:
- `/demo/node-simple.js`:
Simplest possible NodeJS demo for FaceAPI in under 30 lines of JavaScript code
- `/demo/node.js`:
Using `TFJS` native methods to load images without external dependencies
- `/demo/node-canvas.js` and `/demo/node-image.js`:
Using external `canvas` module to load images
Which also allows for image drawing and saving inside `NodeJS` environment
- `/demo/node-match.js`:
Simple demo that compares face similarity from a given image
to a second image or list of images in a folder
- `/demo/node-multiprocess.js`:
Multiprocessing showcase that uses pool of worker processes
(`node-multiprocess-worker.js`)
Main starts fixed pool of worker processes with each worker having
it's instance of `FaceAPI`
Workers communicate with main when they are ready and main dispaches
job to each ready worker until job queue is empty
```json
2021-03-14 08:42:03 INFO: @vladmandic/face-api version 1.0.2
2021-03-14 08:42:03 INFO: User: vlado Platform: linux Arch: x64 Node: v15.7.0
2021-03-14 08:42:03 INFO: FaceAPI multi-process test
2021-03-14 08:42:03 STATE: Main: started worker: 1888019
2021-03-14 08:42:03 STATE: Main: started worker: 1888025
2021-03-14 08:42:04 STATE: Worker: PID: 1888025 TensorFlow/JS 3.3.0 FaceAPI 1.0.2 Backend: tensorflow
2021-03-14 08:42:04 STATE: Worker: PID: 1888019 TensorFlow/JS 3.3.0 FaceAPI 1.0.2 Backend: tensorflow
2021-03-14 08:42:04 STATE: Main: dispatching to worker: 1888019
2021-03-14 08:42:04 STATE: Main: dispatching to worker: 1888025
2021-03-14 08:42:04 DATA: Worker received message: 1888019 { image: 'demo/sample1.jpg' }
2021-03-14 08:42:04 DATA: Worker received message: 1888025 { image: 'demo/sample2.jpg' }
2021-03-14 08:42:06 DATA: Main: worker finished: 1888025 detected faces: 3
2021-03-14 08:42:06 STATE: Main: dispatching to worker: 1888025
2021-03-14 08:42:06 DATA: Worker received message: 1888025 { image: 'demo/sample3.jpg' }
2021-03-14 08:42:06 DATA: Main: worker finished: 1888019 detected faces: 3
2021-03-14 08:42:06 STATE: Main: dispatching to worker: 1888019
2021-03-14 08:42:06 DATA: Worker received message: 1888019 { image: 'demo/sample4.jpg' }
2021-03-14 08:42:07 DATA: Main: worker finished: 1888025 detected faces: 3
2021-03-14 08:42:07 STATE: Main: dispatching to worker: 1888025
2021-03-14 08:42:07 DATA: Worker received message: 1888025 { image: 'demo/sample5.jpg' }
2021-03-14 08:42:08 DATA: Main: worker finished: 1888019 detected faces: 4
2021-03-14 08:42:08 STATE: Main: dispatching to worker: 1888019
2021-03-14 08:42:08 DATA: Worker received message: 1888019 { image: 'demo/sample6.jpg' }
2021-03-14 08:42:09 DATA: Main: worker finished: 1888025 detected faces: 5
2021-03-14 08:42:09 STATE: Main: worker exit: 1888025 0
2021-03-14 08:42:09 DATA: Main: worker finished: 1888019 detected faces: 4
2021-03-14 08:42:09 INFO: Processed 15 images in 5944 ms
2021-03-14 08:42:09 STATE: Main: worker exit: 1888019 0
```
Note that `@tensorflow/tfjs-node` or `@tensorflow/tfjs-node-gpu`
must be installed before using any **NodeJS** examples
## Quick Start
Simply include latest version of `FaceAPI` directly from a CDN in your HTML:
(pick one, `jsdelivr` or `unpkg`)
```html
```
## Installation
`FaceAPI` ships with several pre-build versions of the library:
- `dist/face-api.js`: IIFE format for client-side Browser execution
*with* TFJS pre-bundled
- `dist/face-api.esm.js`: ESM format for client-side Browser execution
*with* TFJS pre-bundled
- `dist/face-api.esm-nobundle.js`: ESM format for client-side Browser execution
*without* TFJS pre-bundled
- `dist/face-api.node.js`: CommonJS format for server-side NodeJS execution
*without* TFJS pre-bundled
- `dist/face-api.node-gpu.js`: CommonJS format for server-side NodeJS execution
*without* TFJS pre-bundled and optimized for CUDA GPU acceleration
Defaults are:
```json
{
"main": "dist/face-api.node-js",
"module": "dist/face-api.esm.js",
"browser": "dist/face-api.esm.js",
}
```
Bundled `TFJS` can be used directly via export: `faceapi.tf`
Reason for additional `nobundle` version is if you want to
include a specific version of TFJS and not rely on pre-packaged one
`FaceAPI` is compatible with TFJS 2.0+ and TFJS 3.0+
All versions include `sourcemap`
There are several ways to use FaceAPI:
### 1. IIFE script
*Recommened for quick tests and backward compatibility with older Browsers that do not support ESM such as IE*
This is simplest way for usage within Browser
Simply download `dist/face-api.js`, include it in your `HTML` file & it's ready to use:
```html
```
IIFE script bundles TFJS and auto-registers global namespace `faceapi` within Window object which can be accessed directly from a `